openEO Platform#
Big Data From Space 2023#
Exploring bottom of atmosphere data
Connect to openEO Platform using python#
import openeo
from openeo.processes import *
conn = openeo.connect("openeo.cloud")
conn = conn.authenticate_oidc()
Authenticated using refresh token.
Look at the collection description#
conn.describe_collection("boa_sentinel_2")
Start creating an openEO process graph#
Pick a spatial extent of interest#
spatial_extent = {'west': 16.2401, 'south': 48.1541, 'east': 16.5595, 'north': 48.2458}
Load the bottom of atmosphere Sentinel 2 collection#
data = conn.load_collection('boa_sentinel_2', spatial_extent = spatial_extent, temporal_extent = ["2020-02-01","2020-12-01"], bands=["B02","B03","B04"])
Aggregate the data for one month using a median#
data_t = data.aggregate_temporal_period(period="month", reducer=median)
Save the result as a netCDF#
saved_data = data_t.save_result(format="NetCDF")
saved_data.flat_graph()
{'loadcollection1': {'process_id': 'load_collection',
'arguments': {'bands': ['B02', 'B03', 'B04'],
'id': 'boa_sentinel_2',
'spatial_extent': {'west': 16.2401,
'south': 48.1541,
'east': 16.5595,
'north': 48.2458},
'temporal_extent': ['2020-02-01', '2020-12-01']}},
'aggregatetemporalperiod1': {'process_id': 'aggregate_temporal_period',
'arguments': {'data': {'from_node': 'loadcollection1'},
'period': 'month',
'reducer': {'process_graph': {'median1': {'process_id': 'median',
'arguments': {'data': {'from_parameter': 'data'}},
'result': True}}}}},
'saveresult1': {'process_id': 'save_result',
'arguments': {'data': {'from_node': 'aggregatetemporalperiod1'},
'format': 'NetCDF',
'options': {}},
'result': True}}
Create and start a job#
job = saved_data.create_job()
job.start_job()
job
Download the results, when the job is finished#
Look at the results using python matplotlib#
from utils import *
path = "./boa/"
output_s2 = load_data(path)
output_s2
<xarray.Dataset> Dimensions: (t: 10, y: 1262, x: 2468) Coordinates: * y (y) float64 1.623e+06 1.623e+06 1.623e+06 ... 1.61e+06 1.61e+06 * x (x) float64 5.26e+06 5.26e+06 5.26e+06 ... 5.285e+06 5.285e+06 spatial_ref int32 0 * t (t) datetime64[ns] 2020-02-29 2020-03-31 ... 2020-11-30 Data variables: B02 (t, y, x) float32 dask.array<chunksize=(1, 1262, 2468), meta=np.ndarray> B03 (t, y, x) float32 dask.array<chunksize=(1, 1262, 2468), meta=np.ndarray> B04 (t, y, x) float32 dask.array<chunksize=(1, 1262, 2468), meta=np.ndarray> Attributes: crs: PROJCS["Azimuthal_Equidistant",GEOGCS["WGS 84",DATUM["WGS_... grid_mapping: spatial_ref nodata: -9999
plt.figure(figsize=(24,24))
plt.subplot(3,1,1)
output = output_s2.isel(t=0)
plt.title("February 2020")
plt.imshow(tone_mapping(output.B04,output.B03,output.B02), cmap='brg')
plt.subplot(3,1,2)
output = output_s2.isel(t=5)
plt.title("July 2020")
plt.imshow(tone_mapping(output.B04,output.B03,output.B02), cmap='brg')
plt.subplot(3,1,3)
output = output_s2.isel(t=9)
plt.title("November 2020")
plt.imshow(tone_mapping(output.B04,output.B03,output.B02), cmap='brg')
<matplotlib.image.AxesImage at 0x7f16e14e84c0>
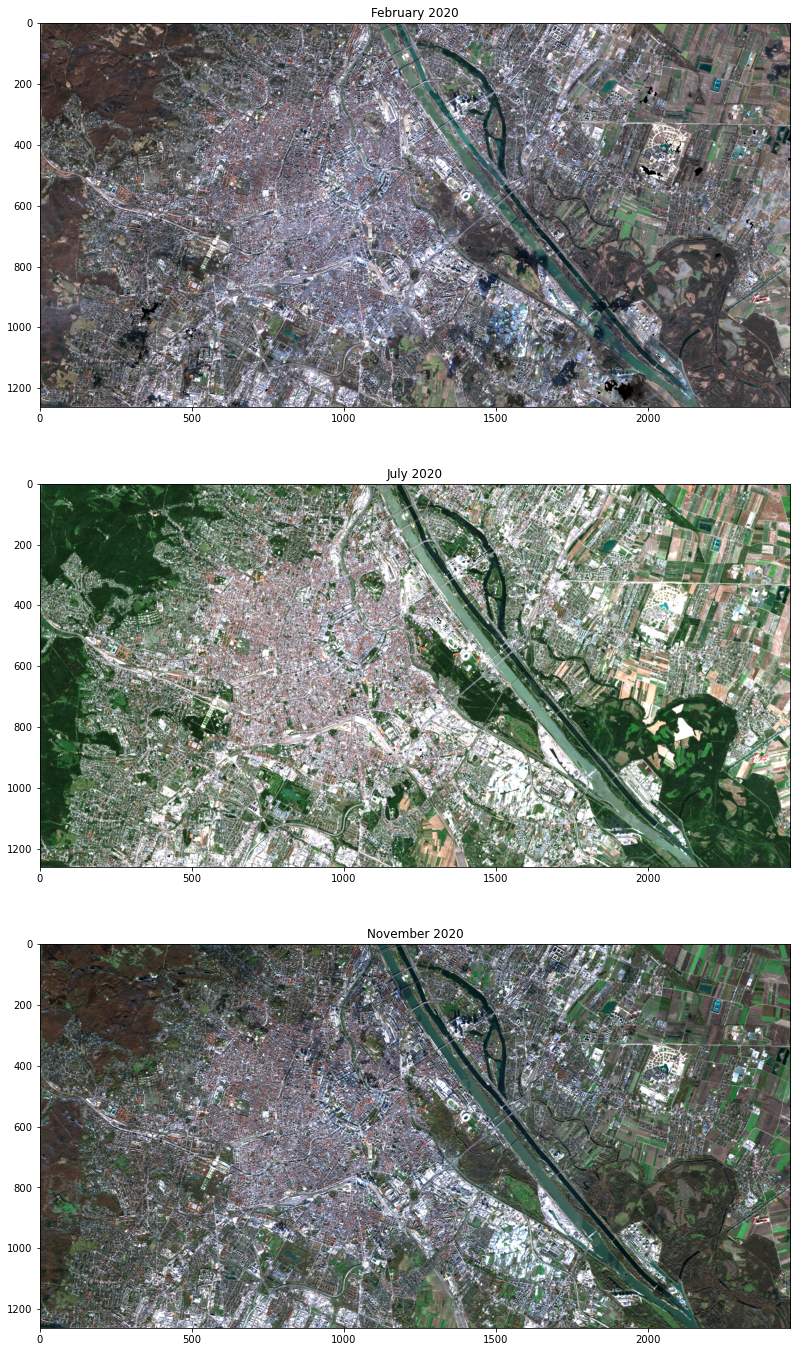